Your cart is currently empty!
Change Woo Add To Cart Button Text & URL Based on Quantity
โ
by
This code changes the add to cart button text on the single product page based on the quantity limit you set per product in the custom field and redirects the user to a custom URL.
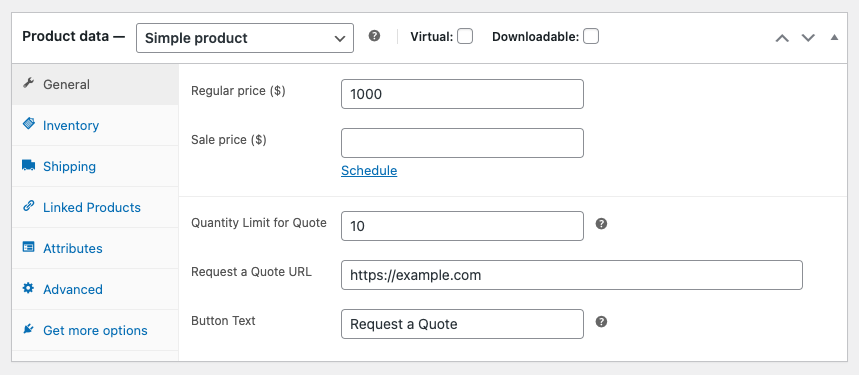
Example : If you set the max quantity to 10 and add a redirect URL to example.com, when the user changes the quantity to 10 or more, the add to cart text for the button changes dynamically and the button URL changes to whatever you set which in this case is example.com.
Demo Video
There’s 3 steps :
Step 1 – Custom Fields
Create 3 custom fields in your child themes functions file for :
- Quantity Limit
- Redirect URL
- Button Text
add_action('woocommerce_product_options_general_product_data', 'wpsitesdotnet_add_custom_fields');
function wpsitesdotnet_add_custom_fields() {
// Custom field for Quantity Limit
woocommerce_wp_text_input(
array(
'id' => '_custom_quantity_limit',
'label' => __('Quantity Limit for Quote', 'woocommerce'),
'desc_tip' => 'true',
'description' => __('Enter the quantity limit for changing the add to cart button to "Request a Quote".', 'woocommerce'),
'type' => 'number',
'custom_attributes' => array(
'step' => '1',
'min' => '0',
),
)
);
// Custom field for Request a Quote URL
woocommerce_wp_text_input(
array(
'id' => '_request_a_quote_url',
'label' => __('Request a Quote URL', 'woocommerce'),
'desc_tip' => 'true',
// 'description' => __('Enter the URL for the Request a Quote page.', 'woocommerce'),
'type' => 'url',
)
);
// Custom field for Request a Quote Button Text
woocommerce_wp_text_input(
array(
'id' => '_request_a_quote_text',
'label' => __('Button Text', 'woocommerce'),
'desc_tip' => 'true',
'description' => __('Enter the text to display for the Request a Quote button.', 'woocommerce'),
'type' => 'text',
'value' => 'Request a Quote'
)
);
}
Step 2 – Save Values
Next we need to save the values added to the fields on the single product admin page :
// Save the custom fields
add_action('woocommerce_admin_process_product_object', 'wpsitesdotnet_save_custom_fields');
function wpsitesdotnet_save_custom_fields($product) {
if (isset($_POST['_custom_quantity_limit'])) {
$quantity_limit = sanitize_text_field($_POST['_custom_quantity_limit']);
$product->update_meta_data('_custom_quantity_limit', $quantity_limit);
}
if (isset($_POST['_request_a_quote_url'])) {
$request_a_quote_url = esc_url_raw($_POST['_request_a_quote_url']);
$product->update_meta_data('_request_a_quote_url', $request_a_quote_url);
}
if (isset($_POST['_request_a_quote_text'])) {
$request_a_quote_text = sanitize_text_field($_POST['_request_a_quote_text']);
$product->update_meta_data('_request_a_quote_text', $request_a_quote_text);
}
}
Step 3 – jQuery Magic
We use jQuery to do 3 things :
- Dynamically change the add to cart button text
- Update button state when quantity reaches the set limit
- Prevent add to cart
- Change add to cart URL to custom redirect URL
Here’s the jQuery you need to make it all work perfectly :
Free
$0
Access only to all free tutorials per month.
Tutorial Request
Includes code guarantee and coding support.
Monthly
$75
Access to 10 premium tutorials per month.
Tutorial Request
Includes code guarantee and coding support.
Yearly
$500
Access to 15 premium tutorials per month.
Monthly Tutorial Request
Includes code guarantee and priority coding support.
Was This Tutorial Helpful?